Building a Chatbot from Scratch with HTML, CSS, and JavaScript
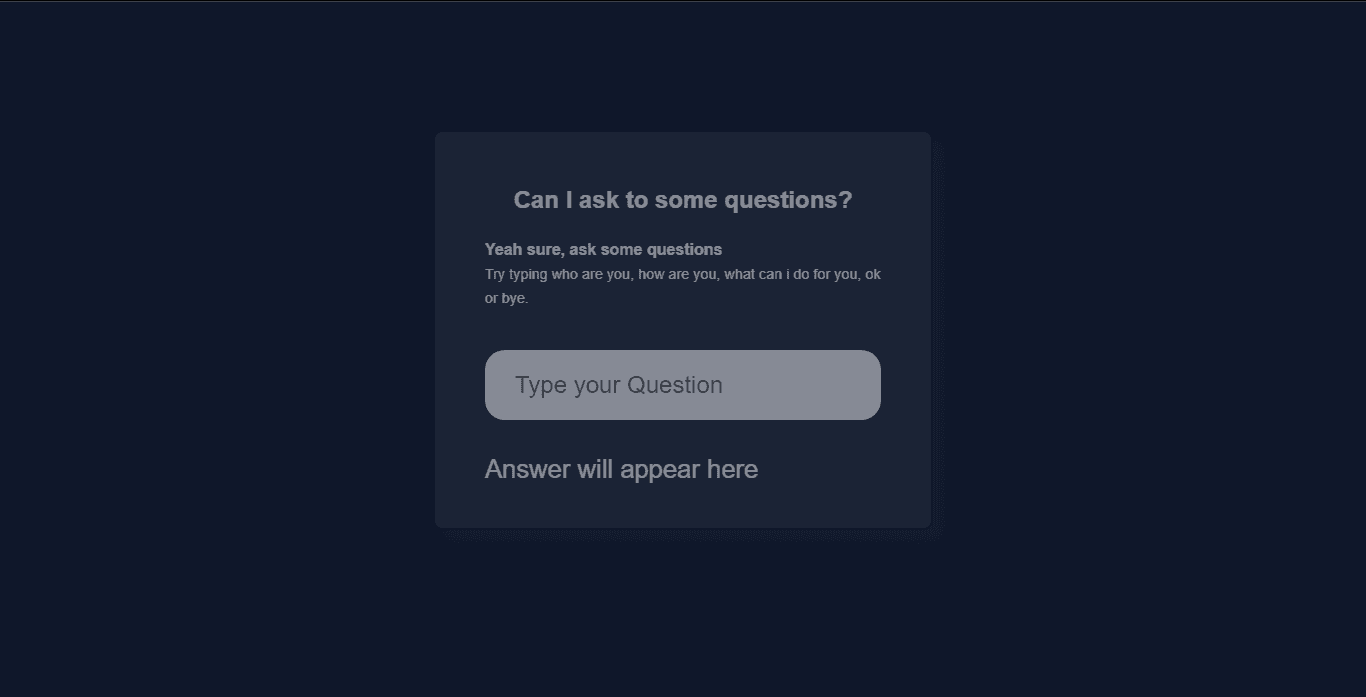
In this tutorial, we'll show you how to create a chatbot using HTML, CSS, and JavaScript. With JavaScript, we can create a chatbot that responds with a specific answer when users enter a specific word.
We'll use HTML to create basic tags such as headings, paragraphs, and an input field for users to type their questions. We'll also style the chatbot's main page using CSS, including the input field where users will type their questions and receive answers.
In terms of HTML, we'll create a container for the chatbot using the div tag, add a heading using the h1 tag, and create an input field using the input tag with the type text attribute. We'll also create a div tag to display the chatbot's answer.
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Chatbot Javascript</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="glass">
<h1>Can I ask to some questions?</h1>
<h2>Yeah sure, ask some questions</h2>
<div class="input">
<input type="text" id="userBox" onkeydown="if(event.keyCode == 13){ talk()}" placeholder="Type your Question" />
</div>
<p id="chatLog">Answer will appear here</p>
</div>
<script src="script.js"></script>
</body>
</html>
Once we've added the HTML structure, we'll add CSS styles to set the margin and padding to zero using the universal tag selector (*), set the box-sizing attribute to border-box, and specify the typeface for the body tag as sans-serif. We'll also set the width and height of the body tag to 100vw and 100vh, respectively. Finally, we'll set the display to flex.
CSS Code:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
width: 100vw;
height: 100vh;
font-family: sans-serif;
padding: 10em 10em;
/* background: url(./bg.jpg); */
background-color: rgb(15 23 42);
opacity: 0.5;
background-position: center;
background-repeat: no-repeat;
background-position: 100% 20%;
background-size: cover;
display: flex;
align-items: center;
justify-content: center;
}
.glass {
width: 500px;
height: 400px;
background-color: rgba(255, 255, 255, 0.1);
padding: 50px;
color: #fff;
border-radius: 9px;
backdrop-filter: blur(50px);
border: 2px solid transparent;
background-clip: padding-box;
box-shadow: 10px 10px 10px rgba(45, 55, 68, 0.3);
line-height: 1.5;
transform: translatey(-5%);
transition: transform 0.5s;
}
.glass-1 {
width: 500px;
height: 400px;
background-color: rgba(255, 255, 255, 0.1);
padding: 50px;
color: rgb(122, 82, 82);
border-radius: 9px;
backdrop-filter: blur(50px);
border: 2px solid transparent;
background-clip: padding-box;
box-shadow: 10px 10px 10px rgba(45, 55, 68, 0.3);
line-height: 1.5;
transform: translatey(-5%);
transition: transform 0.5s;
font-size: 1.7rem;
}
.glass h1 {
font-size: 1.5rem;
text-align: center;
}
.glass h2 {
font-size: 1rem;
margin-top: 20px;
}
.input {
width: 100%;
height: 70px;
overflow: hidden;
margin-top: 40px;
}
.input input {
width: 100%;
height: 70px;
border: none;
padding-left: 30px;
padding-top: 0;
outline: none;
font-size: 1.5rem;
border-radius: 20px;
}
.glass p {
font-size: 1.6rem;
margin-top: 30px;
}
To create the chatbot's functionality, we'll build a function in JavaScript called talk(). Inside the function, we'll create an object variable to store the number of strings before using the document. We'll then choose the HTML element using the getElementById() method and use an if statement to determine whether the user's question matches one stored in the function. If it does, the chatbot will respond with the appropriate answer. Otherwise, the chatbot will display "Sorry, I didn't understand."
JavaScript Code:
function talk() {
var know = {
"who are you": "Hello, Nexcodes here ",
"how are you": "Good :)",
"what can i do for you": "Maybe you can follow me on instagram mrcoder_ig",
"ok": "Thank You So Much ",
"bye": "Okay! Will meet soon..",
};
var user = document.getElementById("userBox").value.toLowerCase();
document.getElementById("chatLog").innerHTML = user + "<br>";
if (user in know) {
document.getElementById("chatLog").innerHTML = know[user] + "<br>";
} else {
document.getElementById("chatLog").innerHTML =
"Sorry,I didn't understand <br>";
}
}
Download Source Code