Neon Navigation Page using Plain Html & CSS
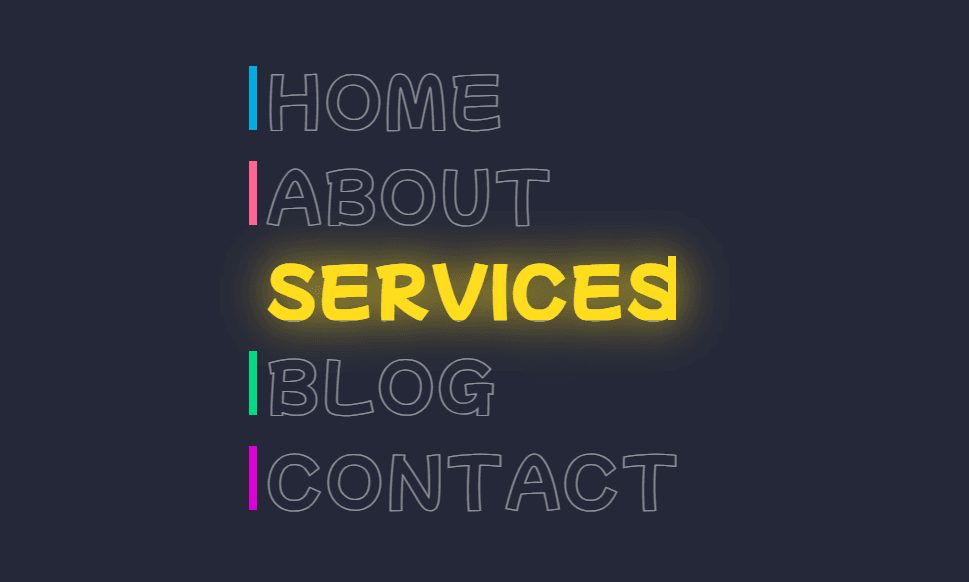
In this tutorial, we'll show you how to create a neon navigation page using pure HTML & CSS. It also contain a cool hover animation with glowing
We'll use HTML to create un-ordered lists with navigation links. We will apply this amazing neon hover effects on those navigation links.
HTML Code:
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Neon Navigation</title>
<link rel="stylesheet" href="./style.css">
</head>
<body>
<ul>
<li style="--clr:#00ade1">
<a href="#" data-text=" Home"> Home </a>
</li>
<li style="--clr:#ff6492">
<a href="#" data-text=" About"> About </a>
</li>
<li style="--clr:#ffdd1c">
<a href="#" data-text=" Services"> Services </a>
</li>
<li style="--clr:#00dc82">
<a href="#" data-text=" Blog"> Blog </a>
</li>
<li style="--clr:#dc00d4">
<a href="#" data-text=" Contact"> Contact </a>
</li>
</ul>
</body>
</html>
- <!DOCTYPE html>: This line specifies the document type and version, indicating that this is an HTML5 document.
- <html lang="en">: The opening <html> tag specifies that this is an HTML document, and the lang attribute sets the language to "en" (English).
- <head>: The <head> element contains meta-information about the document, such as character encoding, title, and links to external resources.
- <meta charset="UTF-8">: This meta tag sets the character encoding to UTF-8, which allows the page to handle a wide range of characters and symbols.
- <title>Neon Navigation</title>: The <title> element sets the title of the webpage, which will be displayed in the browser's title bar or tab.
- <link rel="stylesheet" href="./style.css">: This line links an external CSS file named "style.css" to the HTML document. It allows the HTML document to access the styles defined in the CSS file, helping to separate the content and presentation.
- <body>: The <body> element contains the visible content of the webpage.
- <ul>: This is an unordered list element, used to create a list of items.
- <li style="--clr:#00ade1">: The <li> element represents a list item. The
style
attribute allows setting inline CSS styles for the individual list item. In this case, it sets the variable --clr to the color #00ade1, which is a shade of blue. - <a href="#" data-text=" Home"> Home </a>: The <a> element represents an anchor (hyperlink) and wraps the text "Home." The
href="#"
attribute specifies that it's a placeholder link with no actual destination. The data-text attribute is custom data that will be used later in CSS or JavaScript for styling or interactions. The represents a non-breaking space, adding padding around the word "Home" to create a visual effect. - Similar elements follow for other menu items like "About," "Services," "Blog," and "Contact," each having a different color represented by the --clr variable and the corresponding text wrapped in the anchor tags.
Overall, this code creates a simple navigation menu with visually appealing neon colors for each menu item, achieved through the custom CSS variables and styles applied to each list item. The navigation doesn't have any functionality beyond being visually striking, as the anchor links are set to "#" (a placeholder) and won't lead to any actual pages or sections.
CSS Code:
@import url('https://fonts.googleapis.com/css2?family=Mochiy+Pop+One&display=swap');
* {
box-sizing: border-box;
padding: 0;
margin: 0;
font-family: 'Mochiy Pop One', sans-serif;
}
body {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
background: #252839;
}
ul {
position: relative;
display: flex;
flex-direction: column;
gap: 30px;
}
ul li {
position: relative;
list-style: none;
}
ul li a {
font-size: 4em;
text-decoration: none;
letter-spacing: 2px;
line-height: 1em;
text-transform: uppercase;
color: transparent;
-webkit-text-stroke: 1px rgba(255, 255, 255, 0.5);
}
ul li a::before {
content: attr(data-text);
position: absolute;
color: var(--clr);
width: 0;
overflow: hidden;
transition: 1s;
border-right: 8px solid var(--clr);
-webkit-text-stroke: 1px var(--clr);
}
ul li a:hover::before {
width: 100%;
filter: drop-shadow(0 0 25px var(--clr))
}
@import url('https://fonts.googleapis.com/css2?family=Mochiy+Pop+One&display=swap');
: This line imports the Google Fonts stylesheet for the "Mochiy Pop One" font family. The font will be used throughout the document for all elements since it's defined globally.*
: The asterisk is a CSS universal selector, which means that the following styles will apply to all elements in the document. Here, it setsbox-sizing
toborder-box
, ensures all elements have zero padding, zero margins, and assigns the font family 'Mochiy Pop One', with a fallback tosans-serif
.body
: This selects the<body>
element of the HTML document for styling. It's styled as a flex container, withjustify-content: center
andalign-items: center
to horizontally and vertically center its child elements. Themin-height: 100vh
ensures the body is at least as tall as the viewport, andbackground: #252839
sets a dark grayish-blue background color.ul
: This selects the<ul>
element, which represents the unordered list containing the menu items. It's styled as a flex container with a column layout andgap: 30px
, which creates a 30-pixel gap between each menu item.ul li
: This selects all<li>
elements within the<ul>
element, representing the individual list items in the menu. They are styled withposition: relative
to allow further styling using pseudo-elements (::before and ::after) for the neon effect. Additionally,list-style: none
removes the default bullet points for the list items.ul li a
: This selector targets all anchor (<a>
) elements that are descendants of list item (<li>
) elements, which are themselves descendants of an unordered list (<ul>
). The styles applied to these anchor elements include increasing the font size to 4 times the default size, removing any text decoration (such as underlines), adding 2 pixels of letter spacing between characters, setting the line height to match the font size for vertical centering, transforming the text to uppercase, and making the text color transparent, effectively hiding the anchor text.ul li a::before
: This selector targets the pseudo-element::before
of all anchor (<a>
) elements that are descendants of list item (<li>
) elements, which are themselves descendants of an unordered list (<ul>
). The pseudo-element is used to create the neon effect. It inherits thedata-text
attribute value from the anchor element to display the anchor text content. The pseudo-element is positioned absolutely, and its color is set using the custom CSS variable--clr
, which changes according to the neon color of each menu item. Initially, the width is set to 0, making it invisible, but it creates a neon border on the right side using a solid border and applies a neon-colored text stroke effect.ul li a:hover::before
: This selector targets the pseudo-element::before
of all anchor (<a>
) elements that are descendants of list item (<li>
) elements, which are themselves descendants of an unordered list (<ul>
), but only when the anchor is in a hover state. When hovered, the pseudo-element's width expands to 100%, revealing the neon effect. Additionally, a drop shadow effect is applied with a blur radius of 25 pixels and the neon color (var(--clr)
), resulting in a glowing neon effect on the anchor text when users hover over the menu items. The neon glow is created by the combination of the border and text stroke with the drop shadow effect, giving the appearance of glowing neon text.
Download Source Code