Detect Battery Status using HTML, CSS and JavaScript
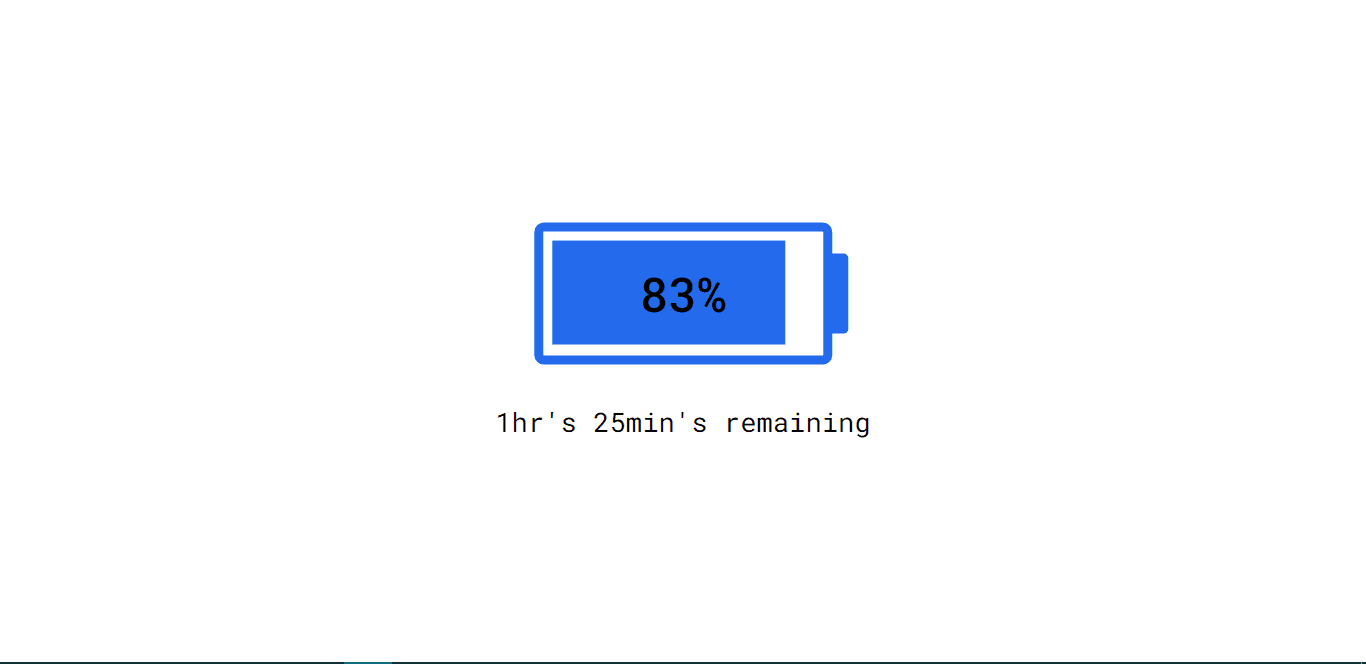
In this tutorial, we'll show you how to create a battery status detector using pure HTML, CSS & JavaScript. It also contain charging animation. it also tell you the remaining battery time.
We'll use HTML to the basic structure of our web page, design it with css & put logic in them using JavaScript 😍
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Detect Battery Status</title>
<!-- Google Fonts -->
<link
href="https://fonts.googleapis.com/css2?family=Roboto+Mono:wght@400;500&display=swap"
rel="stylesheet"
/>
<!-- Stylesheet -->
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="container">
<div id="battery">
<div id="charge"></div>
<div id="charge-level"></div>
</div>
<div id="charging-time"></div>
</div>
<script src="script.js"></script>
</body>
</html>
- <!DOCTYPE html>: This line declares the document type and version as HTML.
- <html lang="en">: This is the opening tag for the HTML document. The lang attribute is set to "en" to specify that the content is in English.
- <head>: This is the head section of the HTML document, which contains meta-information and external resources used by the page.
- <meta name="viewport" content="width=device-width, initial-scale=1.0" />: This meta tag sets the viewport properties for responsive design. It ensures that the width of the page adapts to the device's width and sets the initial scale to 1.0.
- <title>Detect Battery Status</title>: This sets the title of the webpage, which is displayed in the browser's title bar or tab.
- <link href="https://fonts.googleapis.com/css2?family=Roboto+Mono:wght@400;500&display=swap" rel="stylesheet" />: This link tag includes an external stylesheet from Google Fonts. It imports the "Roboto Mono" font in weights 400 and 500 and swaps the display after the font is loaded.
- <link rel="stylesheet" href="style.css" />: This link tag imports an external stylesheet named "style.css" into the HTML document.
- </head>: This is the closing tag for the head section.
- <body>: This is the body section of the HTML document, which contains the visible content of the webpage.
- <div class="container">: This div element with a class of "container" is used to group related elements together.
- <div id="battery">: This div element with an ID of "battery" is used to represent the battery status section.
- <div id="charge"></div>: This div element with an ID of "charge" is likely used to visualize the battery charging status (e.g., using CSS background colors or icons).
- <div id="charge-level"></div>: This div element with an ID of "charge-level" is probably used to display the battery's current charge level (e.g., percentage) using JavaScript or CSS.
- </div>: This is the closing tag for the "battery" div.
- <div id="charging-time"></div>: This div element with an ID of "charging-time" is likely used to display the time remaining for the battery to be fully charged.
- </div>: This is the closing tag for the "container" div.
- <script src="script.js"></script>: This script tag includes an external JavaScript file named "script.js" into the HTML document, probably containing code to detect and update the battery status.
CSS Code:
* {
padding: 0;
margin: 0;
box-sizing: border-box;
font-family: "Roboto Mono", monospace;
}
.container {
position: absolute;
transform: translate(-50%, -50%);
top: 50%;
left: 50%;
}
#battery {
box-sizing: content-box;
height: 7.8em;
width: 17.5em;
border: 0.6em solid #246aed;
margin: auto;
border-radius: 0.6em;
position: relative;
display: grid;
place-items: center;
}
#battery:before {
position: absolute;
content: "";
height: 5em;
width: 1.1em;
background-color: #246aed;
margin: auto;
top: 0;
bottom: 0;
right: -1.6em;
border-radius: 0 0.3em 0.3em 0;
}
#charge {
position: absolute;
height: 6.5em;
width: 16.25em;
background-color: #246aed;
top: 0.6em;
left: 0.6em;
}
#charge-level {
position: absolute;
font-size: 3em;
font-weight: 500;
}
#charging-time {
text-align: center;
font-size: 1.7em;
margin-top: 1.4em;
}
.active {
animation: charge-animation 3s infinite linear;
}
@keyframes charge-animation {
0% {
width: 0;
}
100% {
width: 16.25em;
}
}
- The universal selector * applies the following styles to all elements in the document: padding: 0; sets the padding of all elements to zero, margin: 0; sets the margin of all elements to zero, box-sizing: border-box; ensures that padding and border are included in the element's total width and height, and font-family: "Roboto Mono", monospace; sets the font family to "Roboto Mono" or any monospace font for all elements.
- The .container class styles the container element with the following properties: position: absolute; positions the container absolutely within its nearest positioned ancestor, transform: translate(-50%, -50%); centers the container both horizontally and vertically using the 50% translation trick, and top: 50%; left: 50%; sets the top and left position to 50%, effectively centering the container.
- The #battery ID styles the battery status section with the following properties: box-sizing: content-box; sets the box-sizing to content-box, meaning the width and height include only the content and not padding or border, height: 7.8em; width: 17.5em; sets the height and width of the battery element, border: 0.6em solid #246aed; adds a border around the battery with a color of #246aed, margin: auto; centers the battery element horizontally within its container, border-radius: 0.6em; adds rounded corners to the battery element, position: relative; positions the battery element relative to its normal position, display: grid; place-items: center; centers the content of the battery element using grid layout.
- The #battery:before pseudo-element styles the battery indicator (the vertical line inside the battery) with the following properties: position: absolute; positions the indicator absolutely within the battery element, content: ""; provides empty content for the pseudo-element (used for styling), height: 5em; width: 1.1em; sets the height and width of the indicator, background-color: #246aed; sets the background color of the indicator to #246aed (matching the battery border color), margin: auto; centers the indicator horizontally within its parent (battery) element, top: 0; bottom: 0; right: -1.6em; positions the indicator to the right side of the battery element, border-radius: 0 0.3em 0.3em 0; adds a rounded end to the indicator to match the battery shape.
- The #charge ID styles the battery charge level indicator with the following properties: position: absolute; positions the charge level indicator absolutely within the battery element, height: 6.5em; width: 16.25em; sets the height and width of the charge level indicator, background-color: #246aed; sets the background color of the charge level indicator to #246aed (matching the battery border color), top: 0.6em; left: 0.6em; adds a small margin from the top and left sides, separating the charge level indicator from the battery border.
- The #charge-level ID styles the text that displays the battery charge level with the following properties: position: absolute; positions the charge level text absolutely within the battery element, font-size: 3em; sets the font size to 3em for the charge level text, font-weight: 500; sets the font weight to 500 (semi-bold) for the charge level text.
- The #charging-time ID styles the text that displays the charging time with the following properties: text-align: center; centers the charging time text within its container, font-size: 1.7em; sets the font size to 1.7em for the charging time text, margin-top: 1.4em; adds a top margin to separate the charging time text from the battery charge level indicator.
- The .active class styles an element with the "active" class, which is likely applied dynamically using JavaScript: animation: charge-animation 3s infinite linear; applies the "charge-animation" animation to the element with the "active" class.
- The @keyframes charge-animation defines the "charge-animation" animation, which animates the width of the element from 0 to 16.25em over a period of 3 seconds in a linear manner. This animation is used to visually represent the charging progress.
JavaScript Code:
const chargeLevel = document.getElementById("charge-level");
const charge = document.getElementById("charge");
const chargingTimeRef = document.getElementById("charging-time");
window.onload = () => {
//For browsers that don't support the battery status API
if (!navigator.getBattery) {
alert("Battery Status Api Is Not Supported In Your Browser");
return false;
}
};
navigator.getBattery().then((battery) => {
function updateAllBatteryInfo() {
updateChargingInfo();
updateLevelInfo();
}
updateAllBatteryInfo();
//When the charging status changes
battery.addEventListener("chargingchange", () => {
updateAllBatteryInfo();
});
//When the Battery Levvel Changes
battery.addEventListener("levelchange", () => {
updateAllBatteryInfo();
});
function updateChargingInfo() {
if (battery.charging) {
charge.classList.add("active");
chargingTimeRef.innerText = "";
} else {
charge.classList.remove("active");
//Display time left to discharge only when it is a integer value i.e not infinity
if (parseInt(battery.dischargingTime)) {
let hr = parseInt(battery.dischargingTime / 3600);
let min = parseInt(battery.dischargingTime / 60 - hr * 60);
chargingTimeRef.innerText = `${hr}hr's ${min}min's remaining`;
}
}
}
//Updating battery level
function updateLevelInfo() {
let batteryLevel = `${parseInt(battery.level * 100)}%`;
charge.style.width = batteryLevel;
chargeLevel.textContent = batteryLevel;
}
});
- Three constant variables, chargeLevel, charge, and chargingTimeRef, are declared using const, representing references to specific elements in the HTML document with the IDs "charge-level", "charge", and "charging-time", respectively. These variables will be used to update and display battery-related information on the webpage.
- The window.onload event is set to an arrow function. This function executes when the webpage finishes loading. Inside the function, a check is performed to see if the browser supports the Battery Status API using navigator.getBattery.
- If the browser does not support the Battery Status API (when navigator.getBattery is not available), an alert message is displayed to inform the user that the API is not supported in their browser. The function then returns false.
- After the webpage has loaded and if the browser supports the Battery Status API, the navigator.getBattery().then((battery) => { ... }) function is called to obtain the battery object and perform actions based on the battery status.
- Inside the then() block, a function called updateAllBatteryInfo is defined. This function serves the purpose of updating both the charging information and battery level information on the webpage.
- The updateAllBatteryInfo function is immediately called once after obtaining the battery object to initialize the displayed battery status and charge level.
- Two event listeners are attached to the battery object to monitor changes in the battery status. The first event listener, battery.addEventListener("chargingchange", () => { ... }), is triggered whenever the charging status of the battery changes. When this event occurs, it calls the updateAllBatteryInfo function to update the displayed charging information and battery level on the webpage. This ensures that any changes in the charging status are immediately reflected in the user interface, allowing users to stay informed about their device's charging progress.
- Similarly, the second event listener, battery.addEventListener("levelchange", () => { ... }), is activated whenever the battery level changes. Like the first listener, it also calls the updateAllBatteryInfo function, ensuring that any adjustments to the battery level are immediately updated in the displayed charging information and battery level on the webpage. This real-time update helps users keep track of their device's battery level, enabling them to make informed decisions about charging or using their device efficiently. By utilizing these event listeners, the webpage remains up-to-date with the latest battery status, providing a seamless and responsive user experience.
- The updateChargingInfo function plays a crucial role in updating the charging information based on the battery's current charging status. When the battery is actively charging (battery.charging is true), the function adds the "active" class to the charge element. This class likely triggers a CSS animation that visually indicates the device is currently charging, providing users with a clear visual cue. Furthermore, during the charging process, the content of the chargingTimeRef element is set to an empty string, ensuring that no remaining time text is displayed, as it would not be relevant while the battery is charging.
- Conversely, if the battery is not charging (when battery.charging is false), the updateChargingInfo function removes the "active" class from the charge element, effectively stopping the CSS animation. Additionally, the function checks if the battery.dischargingTime value is a valid integer (not infinity). If it is valid, the function calculates the remaining hours and minutes until the battery is fully discharged. The calculated time is then updated in the chargingTimeRef element, providing users with an estimated time left until the battery is completely depleted. This gives users valuable information about their device's power status, enabling them to plan their usage accordingly.
- On the other hand, the updateLevelInfo function is responsible for handling the updating of the displayed battery level information. First, it calculates the current battery level as a percentage by multiplying battery.level by 100 and creates a string with the percentage value. This calculated battery level is then used to adjust the width of the
charge
element using CSS, visually representing the battery charge level to the user. By dynamically adjusting the width, the battery level is intuitively displayed in a visually appealing manner. - Moreover, the function updates the text content of the chargeLevel element to display the current battery level in percentage format to the user. This textual representation of the battery level complements the visual representation provided by the charge element's width, ensuring users have clear and concise information about their device's battery level at a glance. By combining both visual and textual feedback, users can quickly assess their device's battery status and make informed decisions about charging or using their device efficiently. Overall, the updateChargingInfo and updateLevelInfo functions work together to provide users with accurate and real-time battery status information, enhancing the user experience and usability of the webpage.
Download Source Code