Create an Alarm Clock using Html, Css & Javascript only
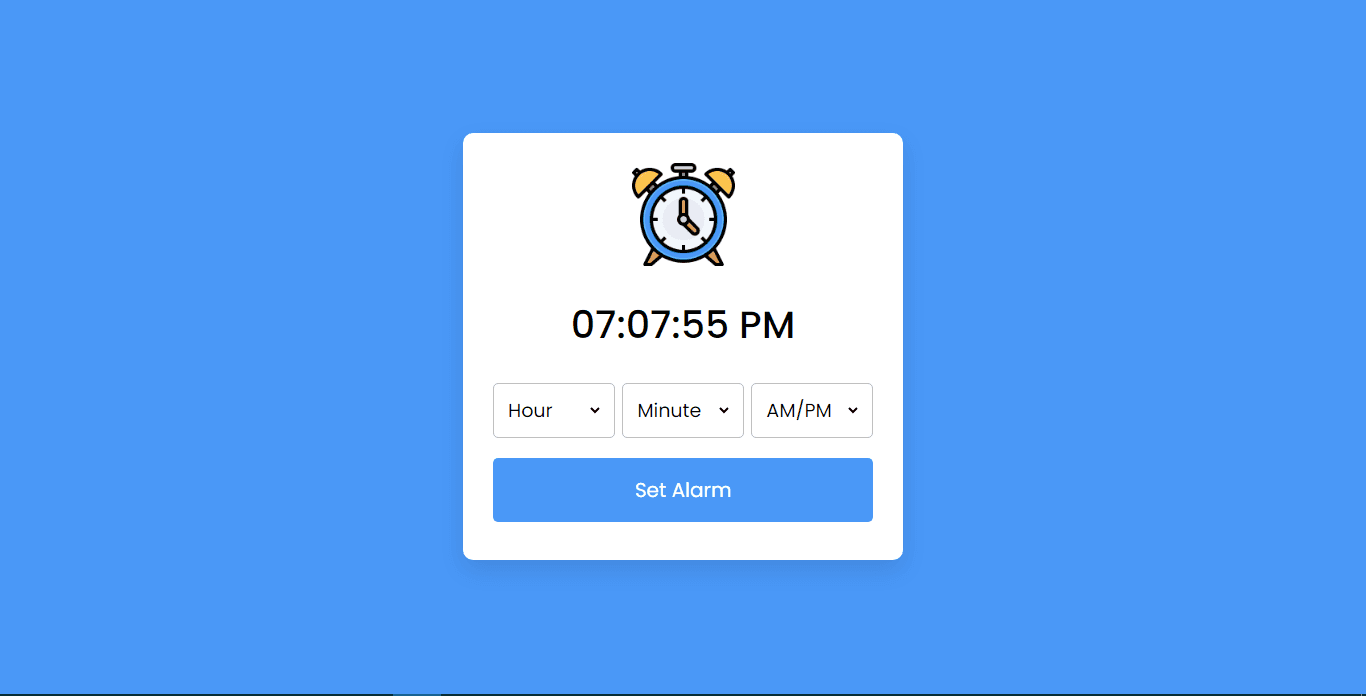
In this tutorial, we will explore how to create an alarm clock using HTML, CSS, and JavaScript. An alarm clock is a useful tool that helps us wake up on time, manage our schedules, and maintain a healthy sleep pattern. By building an alarm clock using web technologies, we can create a customizable and portable solution that can be easily integrated into websites or web applications.
We will begin by constructing the basic structure of the alarm clock using HTML, which will include elements such as the current time display, alarm input, and alarm status. Next, we will apply CSS to style the alarm clock, making it visually appealing and user-friendly. Finally, we will implement the alarm functionality using JavaScript, allowing users to set alarms and receive notifications when the alarm time is reached.
Throughout this tutorial, we will cover various HTML, CSS, and JavaScript concepts, such as working with the Date object, handling user input, and manipulating the DOM. By the end of this tutorial, you will have a fully functional alarm clock that can be easily integrated into your projects or used as a standalone application.
So, let's get started and create an alarm clock using HTML, CSS, and JavaScript!
HTML Code:
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Alarm Clock in JavaScript</title>
<link rel="stylesheet" href="style.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<div class="wrapper">
<img src="./files/clock.svg" alt="clock">
<h1>00:00:00 PM</h1>
<div class="content">
<div class="column">
<select>
<option value="Hour" selected disabled hidden>Hour</option>
</select>
</div>
<div class="column">
<select>
<option value="Minute" selected disabled hidden>Minute</option>
</select>
</div>
<div class="column">
<select>
<option value="AM/PM" selected disabled hidden>AM/PM</option>
</select>
</div>
</div>
<button>Set Alarm</button>
</div>
<script src="script.js"></script>
</body>
</html>
This is an HTML code snippet for creating an alarm clock web page. Here is a breakdown of the code:
<!DOCTYPE html>
: This line declares that the document is an HTML document.
<html lang="en" dir="ltr">
: This line is the opening tag for the HTML document. The lang
attribute specifies the language of the document, and the dir
attribute specifies the direction of the text.
<head>
: This tag contains the head section of the HTML document. This section typically contains meta information, such as the page title, links to CSS files, and scripts.
<meta charset="utf-8">
: This tag specifies the character encoding for the document, which is utf-8.
<title>Alarm Clock in JavaScript</title>
: This tag specifies the title of the web page that will be displayed in the browser tab.
<link rel="stylesheet" href="style.css">
: This tag specifies the link to an external CSS file that contains the styling information for the web page.
<meta name="viewport" content="width=device-width, initial-scale=1.0">
: This tag specifies the viewport of the document for mobile devices.
<body>
: This tag contains the body section of the HTML document. This section typically contains the content of the web page.
<div class="wrapper">
: This tag creates a container for the content of the web page. The class
attribute specifies the CSS class that will be used to style this element.
<img src="./files/clock.svg" alt="clock">
: This tag creates an image element that displays a clock icon. The src
attribute specifies the location of the image file, and the alt
attribute specifies the text that will be displayed if the image cannot be loaded.
<h1>00:00:00 PM</h1>
: This tag creates a heading element that displays the current time in 12-hour format.
<div class="content">
: This tag creates a container for the alarm settings.
<div class="column">
: This tag creates a container for the hour select element.
<select>
: This tag creates a select element that allows the user to choose the hour for the alarm.
<option value="Hour" selected disabled hidden>Hour</option>
: This tag creates an option element that specifies the default value for the hour select element.
<div class="column">
: This tag creates a container for the minute select element.
<select>
: This tag creates a select element that allows the user to choose the minute for the alarm.
<option value="Minute" selected disabled hidden>Minute</option>
: This tag creates an option element that specifies the default value for the minute select element.
<div class="column">
: This tag creates a container for the AM/PM select element.
<select>
: This tag creates a select element that allows the user to choose between AM and PM for the alarm time.
<option value="AM/PM" selected disabled hidden>AM/PM</option>
: This tag creates an option element that specifies the default value for the AM/PM select element.
<button>Set Alarm</button>
: This tag creates a button element that allows the user to set the alarm.
<script src="script.js"></script>
: This tag specifies the link to an external JavaScript file that contains the logic for the alarm clock.
CSS Code:
/* Import Google font - Poppins */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body, .wrapper, .content{
display: flex;
align-items: center;
justify-content: center;
}
body{
padding: 0 10px;
min-height: 100vh;
background: #4A98F7;
}
.wrapper{
width: 440px;
padding: 30px 30px 38px;
background: #fff;
border-radius: 10px;
flex-direction: column;
box-shadow: 0 10px 25px rgba(0,0,0,0.1);
}
.wrapper img{
max-width: 103px;
}
.wrapper h1{
font-size: 38px;
font-weight: 500;
margin: 30px 0;
}
.wrapper .content{
width: 100%;
justify-content: space-between;
}
.content.disable{
cursor: no-drop;
}
.content .column{
padding: 0 10px;
border-radius: 5px;
border: 1px solid #bfbfbf;
width: calc(100% / 3 - 5px);
}
.content.disable .column{
opacity: 0.6;
pointer-events: none;
}
.column select{
width: 100%;
height: 53px;
border: none;
outline: none;
background: none;
font-size: 19px;
}
.wrapper button{
width: 100%;
border: none;
outline: none;
color: #fff;
cursor: pointer;
font-size: 20px;
padding: 17px 0;
margin-top: 20px;
border-radius: 5px;
background: #4A98F7;
}
- The code imports a Google font called Poppins and sets some global CSS styles with the universal selector
*
. These styles include setting margins and padding to 0, the box-sizing to border-box, and the font family to Poppins. - The
body
,.wrapper
, and.content
selectors all havedisplay: flex
,align-items: center
, andjustify-content: center
properties set. Thebody
selector also sets a background color of #4A98F7 and a minimum height of 100vh, while the.wrapper
selector sets a width of 440px, padding, background color, and box-shadow properties. - The
.wrapper img
selector sets a max-width for the image within.wrapper
, while the.wrapper h1
selector sets font-size and font-weight properties. The.wrapper .content
selector sets width and justify-content properties, while the.content.disable
selector sets a cursor property. - The
.content .column
selector sets padding, border-radius, border, and width properties for the.column
class within.content
. The.content.disable .column
selector sets opacity and pointer-events properties. The.column select
selector sets width, height, and font-size properties for theselect
element within.column
. - The
.wrapper button
selector sets several button properties including width, border, color, cursor, font-size, padding, margin-top, border-radius, and background.
JavaScript Code:
const currentTime = document.querySelector("h1"),
content = document.querySelector(".content"),
selectMenu = document.querySelectorAll("select"),
setAlarmBtn = document.querySelector("button");
let alarmTime, isAlarmSet,
ringtone = new Audio("./files/ringtone.mp3");
for (let i = 12; i > 0; i--) {
i = i < 10 ? `0${i}` : i;
let option = `<option value="${i}">${i}</option>`;
selectMenu[0].firstElementChild.insertAdjacentHTML("afterend", option);
}
for (let i = 59; i >= 0; i--) {
i = i < 10 ? `0${i}` : i;
let option = `<option value="${i}">${i}</option>`;
selectMenu[1].firstElementChild.insertAdjacentHTML("afterend", option);
}
for (let i = 2; i > 0; i--) {
let ampm = i == 1 ? "AM" : "PM";
let option = `<option value="${ampm}">${ampm}</option>`;
selectMenu[2].firstElementChild.insertAdjacentHTML("afterend", option);
}
setInterval(() => {
let date = new Date(),
h = date.getHours(),
m = date.getMinutes(),
s = date.getSeconds(),
ampm = "AM";
if(h >= 12) {
h = h - 12;
ampm = "PM";
}
h = h == 0 ? h = 12 : h;
h = h < 10 ? "0" + h : h;
m = m < 10 ? "0" + m : m;
s = s < 10 ? "0" + s : s;
currentTime.innerText = `${h}:${m}:${s} ${ampm}`;
if (alarmTime === `${h}:${m} ${ampm}`) {
ringtone.play();
ringtone.loop = true;
}
});
function setAlarm() {
if (isAlarmSet) {
alarmTime = "";
ringtone.pause();
content.classList.remove("disable");
setAlarmBtn.innerText = "Set Alarm";
return isAlarmSet = false;
}
let time = `${selectMenu[0].value}:${selectMenu[1].value} ${selectMenu[2].value}`;
if (time.includes("Hour") || time.includes("Minute") || time.includes("AM/PM")) {
return alert("Please, select a valid time to set Alarm!");
}
alarmTime = time;
isAlarmSet = true;
content.classList.add("disable");
setAlarmBtn.innerText = "Clear Alarm";
}
setAlarmBtn.addEventListener("click", setAlarm);
This code written in JavaScript contains the following functionalities:
- It selects HTML elements using
document.querySelector
anddocument.querySelectorAll
methods and stores their reference in constantscurrentTime
,content
,selectMenu
, andsetAlarmBtn
. - It creates an instance of the
Audio
object and loads an audio file into it, which will be played when the alarm time matches the current time. - It uses
for
loops to dynamically create options for the select dropdowns for hours, minutes, and AM/PM. - It sets an interval using the
setInterval
method to update the clock time displayed inh1
tag, and checks if the alarm time has been set and if it matches the current time. If it does, it plays the ringtone audio file. - It defines a
setAlarm
function, which sets or clears the alarm time based on the state of theisAlarmSet
variable. When the alarm is set, it disables thecontent
element, changes the text ofsetAlarmBtn
to "Clear Alarm", and setsalarmTime
to the selected time. When the alarm is cleared, it enables thecontent
element, changes the text ofsetAlarmBtn
to "Set Alarm", and clears thealarmTime
. - It adds an event listener to the
setAlarmBtn
element that calls thesetAlarm
function when it is clicked.
Download Source Code